So I was watching some memes on r/formuladank (the only good thing reddit is good for anyway), and I stumbled upon this little gem:
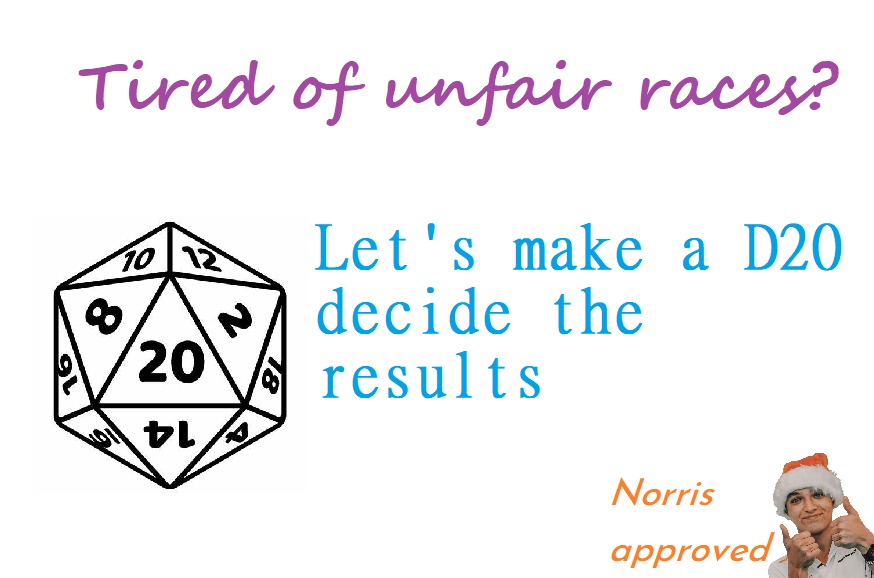
This made me think: what would happen if we’d have actually a D20 roll for each championship?
So without further ado, while I was waiting in the airport for my plane, I started to write the code. I turned to Wikipedia to get the list of the drivers enlisted by the teams this year and then also wrote a small piece of code to exclude various driver line-up changes.
|
|
This piece of code creates a simple table and pulls one random driver which it deems the winner of said race and puts it into a table along with how many wins he has. It’s all random (as much as PHP randomness is random) and it doesn’t take into account chaos events like weather, crashes, etc, because that would be a lot of statistic data that I don’t want to process. The result looks like this:
Race | Driver | Wins |
---|---|---|
Bahrain Grand Prix | Nico HĂŒlkenberg | (1) |
Saudi Arabian Grand Prix | Daniel Ricciardo | (1) |
Australian Grand Prix | Kevin Magnussen | (1) |
Japanese Grand Prix | Zhou Guanyu | (1) |
Chinese Grand Prix | Lance Stroll | (1) |
Miami Grand Prix | Sergio Pérez | (1) |
Emilia Romagna Grand Prix | Zhou Guanyu | (2) |
Monaco Grand Prix | Zhou Guanyu | (3) |
Canadian Grand Prix | Oscar Piastri | (1) |
Spanish Grand Prix | Max Verstappen | (1) |
Austrian Grand Prix | Pierre Gasly | (1) |
British Grand Prix | Yuki Tsunoda | (1) |
Hungarian Grand Prix | Valtteri Bottas | (1) |
Belgian Grand Prix | Lando Norris | (1) |
Dutch Grand Prix | Esteban Ocon | (1) |
Italian Grand Prix | Franco Colapinto | (1) |
Azerbaijan Grand Prix | Max Verstappen | (2) |
Singapore Grand Prix | Zhou Guanyu | (4) |
United States Grand Prix | Nico HĂŒlkenberg | (2) |
Mexico City Grand Prix | Franco Colapinto | (2) |
SĂŁo Paulo Grand Prix | Kevin Magnussen | (2) |
Las Vegas Grand Prix | Sergio Pérez | (2) |
Qatar Grand Prix | Esteban Ocon | (2) |
Abu Dhabi Grand Prix | Fernando Alonso | (1) |
|
|
After all this process, I add the replacement drivers to the list, and then I sort the winners total table so we can see which driver had the most wins over the 24 races and place it in a table next to the number of winners.
Number of winners: 15
Driver | Wins |
---|---|
24. Zhou Guanyu | 4 |
27. Nico HĂŒlkenberg | 2 |
20. Kevin Magnussen | 2 |
11. Sergio Pérez | 2 |
1. Max Verstappen | 2 |
31. Esteban Ocon | 2 |
43. Franco Colapinto | 2 |
3. Daniel Ricciardo | 1 |
18. Lance Stroll | 1 |
81. Oscar Piastri | 1 |
10. Pierre Gasly | 1 |
22. Yuki Tsunoda | 1 |
77. Valtteri Bottas | 1 |
4. Lando Norris | 1 |
14. Fernando Alonso | 1 |
Jumping to conclusions
Using the meme as a starting point for this small project, it seems that on my test, we’re having about 12-16 winners every run of the script, and sometimes the championship is tied in number of victories. Funny enough, in a batch of 10 tests, Zhou Guanyu won the championship twice, Oscar Piastri once and Lando Norris once as well (but after Oscar, lmao).
If you want to play yourself with this, you can follow the tool available here: Formula 1 Season Simple Random Simulator
The Advanced Formula 1 Random Simulator
Having so many ties (I had one run with 17 winners and a six-way championship tie!) quickly prompted me to develop more this script and make it take into account points awarded for running the entire race. There are some less points awarded if the race has to be shorter (many red flags, bad weather, etc), but these situation happen so rarely, I’m not taking them into account. As Formula 1 states:
The scoring system awards championship points for the first ten finishers at each Grand Prix, with 25 points for the winner, before scaling down to 18, 15, 12, 10, 8, 6, 4, 2, and a single point for the 10th-placed driver. Thereâs an additional point available for the driver with the fastest lap of the race (if they finish in the top ten), plus more points available for success in F1 Sprint races. The driver with the most points at the end of the year wins the title. No end of season play-offs.
Also for the sake of simplifying the code, I will not take into account neither sprints nor fastest lap, and the championship will be decided only by the race points.
Converting the code of the simple generator to the new one, was fairly simple, instead of using a random pull from the drivers array to decide the winner, I had to iterate over an array of points assignment
|
|
The results can be seen easily in the table below (you need to click on the plus sign to the right to expand the table), I’ve added in the brackets the total points of that driver after the respective race.
Year Races
Race | Driver | Wins |
---|---|---|
Bahrain Grand Prix | 1. Carlos Sainz Jr. | (25) |
2. Logan Sargeant | (18) | |
3. Zhou Guanyu | (15) | |
4. Kevin Magnussen | (12) | |
5. Lewis Hamilton | (10) | |
6. Alexander Albon | (8) | |
7. Sergio Pérez | (6) | |
8. George Russell | (4) | |
9. Daniel Ricciardo | (2) | |
10. Yuki Tsunoda | (1) | |
Saudi Arabian Grand Prix | 1. Pierre Gasly | (25) |
2. Daniel Ricciardo | (20) | |
3. Logan Sargeant | (33) | |
4. Max Verstappen | (12) | |
5. Esteban Ocon | (10) | |
6. Fernando Alonso | (8) | |
7. Charles Leclerc | (6) | |
8. Zhou Guanyu | (19) | |
9. Alexander Albon | (10) | |
10. George Russell | (5) | |
Australian Grand Prix | 1. Valtteri Bottas | (25) |
2. Pierre Gasly | (43) | |
3. Max Verstappen | (27) | |
4. George Russell | (17) | |
5. Yuki Tsunoda | (11) | |
6. Lewis Hamilton | (18) | |
7. Daniel Ricciardo | (26) | |
8. Logan Sargeant | (37) | |
9. Kevin Magnussen | (14) | |
10. Fernando Alonso | (9) | |
Japanese Grand Prix | 1. Kevin Magnussen | (39) |
2. Charles Leclerc | (24) | |
3. Lando Norris | (15) | |
4. Carlos Sainz Jr. | (37) | |
5. Fernando Alonso | (19) | |
6. Logan Sargeant | (45) | |
7. Nico HĂŒlkenberg | (6) | |
8. Max Verstappen | (31) | |
9. Lance Stroll | (2) | |
10. Zhou Guanyu | (20) | |
Chinese Grand Prix | 1. Pierre Gasly | (68) |
2. Nico HĂŒlkenberg | (24) | |
3. Yuki Tsunoda | (26) | |
4. Zhou Guanyu | (32) | |
5. Lance Stroll | (12) | |
6. Valtteri Bottas | (33) | |
7. Alexander Albon | (16) | |
8. Esteban Ocon | (14) | |
9. Charles Leclerc | (26) | |
10. Daniel Ricciardo | (27) | |
Miami Grand Prix | 1. Daniel Ricciardo | (52) |
2. Yuki Tsunoda | (44) | |
3. Valtteri Bottas | (48) | |
4. Kevin Magnussen | (51) | |
5. Max Verstappen | (41) | |
6. Lewis Hamilton | (26) | |
7. Sergio Pérez | (12) | |
8. Lando Norris | (19) | |
9. Alexander Albon | (18) | |
10. Lance Stroll | (13) | |
Emilia Romagna Grand Prix | 1. Lance Stroll | (38) |
2. George Russell | (35) | |
3. Kevin Magnussen | (66) | |
4. Max Verstappen | (53) | |
5. Sergio Pérez | (22) | |
6. Lando Norris | (27) | |
7. Fernando Alonso | (25) | |
8. Daniel Ricciardo | (56) | |
9. Logan Sargeant | (47) | |
10. Lewis Hamilton | (27) | |
Monaco Grand Prix | 1. Charles Leclerc | (51) |
2. Sergio Pérez | (40) | |
3. George Russell | (50) | |
4. Franco Colapinto | (12) | |
5. Max Verstappen | (63) | |
6. Lewis Hamilton | (35) | |
7. Esteban Ocon | (20) | |
8. Valtteri Bottas | (52) | |
9. Oscar Piastri | (2) | |
10. Fernando Alonso | (26) | |
Canadian Grand Prix | 1. Kevin Magnussen | (91) |
2. Sergio Pérez | (58) | |
3. Daniel Ricciardo | (71) | |
4. Fernando Alonso | (38) | |
5. Oscar Piastri | (12) | |
6. Lando Norris | (35) | |
7. Lance Stroll | (44) | |
8. Alexander Albon | (22) | |
9. George Russell | (52) | |
10. Logan Sargeant | (48) | |
Spanish Grand Prix | 1. Alexander Albon | (47) |
2. Carlos Sainz Jr. | (55) | |
3. Franco Colapinto | (27) | |
4. Logan Sargeant | (60) | |
5. Charles Leclerc | (61) | |
6. Fernando Alonso | (46) | |
7. Lando Norris | (41) | |
8. Pierre Gasly | (72) | |
9. Sergio Pérez | (60) | |
10. Kevin Magnussen | (92) | |
Austrian Grand Prix | 1. Fernando Alonso | (71) |
2. Pierre Gasly | (90) | |
3. Oscar Piastri | (27) | |
4. Carlos Sainz Jr. | (67) | |
5. Zhou Guanyu | (42) | |
6. Esteban Ocon | (28) | |
7. Nico HĂŒlkenberg | (30) | |
8. George Russell | (56) | |
9. Valtteri Bottas | (54) | |
10. Alexander Albon | (48) | |
British Grand Prix | 1. Nico HĂŒlkenberg | (55) |
2. Valtteri Bottas | (72) | |
3. Pierre Gasly | (105) | |
4. Sergio Pérez | (72) | |
5. Lance Stroll | (54) | |
6. Lewis Hamilton | (43) | |
7. George Russell | (62) | |
8. Logan Sargeant | (64) | |
9. Max Verstappen | (65) | |
10. Daniel Ricciardo | (72) | |
Hungarian Grand Prix | 1. Lando Norris | (66) |
2. Sergio Pérez | (90) | |
3. Carlos Sainz Jr. | (82) | |
4. Alexander Albon | (60) | |
5. Zhou Guanyu | (52) | |
6. Esteban Ocon | (36) | |
7. Lewis Hamilton | (49) | |
8. Charles Leclerc | (65) | |
9. Yuki Tsunoda | (46) | |
10. Fernando Alonso | (72) | |
Belgian Grand Prix | 1. Esteban Ocon | (61) |
2. Max Verstappen | (83) | |
3. Charles Leclerc | (80) | |
4. Valtteri Bottas | (84) | |
5. George Russell | (72) | |
6. Lewis Hamilton | (57) | |
7. Kevin Magnussen | (98) | |
8. Yuki Tsunoda | (50) | |
9. Carlos Sainz Jr. | (84) | |
10. Sergio Pérez | (91) | |
Dutch Grand Prix | 1. Lewis Hamilton | (82) |
2. Carlos Sainz Jr. | (102) | |
3. Kevin Magnussen | (113) | |
4. Valtteri Bottas | (96) | |
5. George Russell | (82) | |
6. Nico HĂŒlkenberg | (63) | |
7. Esteban Ocon | (67) | |
8. Sergio Pérez | (95) | |
9. Oscar Piastri | (29) | |
10. Zhou Guanyu | (53) | |
Italian Grand Prix | 1. Kevin Magnussen | (138) |
2. Daniel Ricciardo | (90) | |
3. Zhou Guanyu | (68) | |
4. Valtteri Bottas | (108) | |
5. Carlos Sainz Jr. | (112) | |
6. Lance Stroll | (62) | |
7. Oscar Piastri | (35) | |
8. Lando Norris | (70) | |
9. Esteban Ocon | (69) | |
10. Pierre Gasly | (106) | |
Azerbaijan Grand Prix | 1. Sergio Pérez | (120) |
2. Pierre Gasly | (124) | |
3. Esteban Ocon | (84) | |
4. George Russell | (94) | |
5. Zhou Guanyu | (78) | |
6. Lando Norris | (78) | |
7. Franco Colapinto | (33) | |
8. Oliver Bearman | (4) | |
9. Lewis Hamilton | (84) | |
10. Alexander Albon | (61) | |
Singapore Grand Prix | 1. Charles Leclerc | (105) |
2. Nico HĂŒlkenberg | (81) | |
3. Franco Colapinto | (48) | |
4. Valtteri Bottas | (120) | |
5. Fernando Alonso | (82) | |
6. Esteban Ocon | (92) | |
7. Max Verstappen | (89) | |
8. George Russell | (98) | |
9. Carlos Sainz Jr. | (114) | |
10. Sergio Pérez | (121) | |
United States Grand Prix | 1. Sergio Pérez | (146) |
2. Alexander Albon | (79) | |
3. Franco Colapinto | (63) | |
4. Carlos Sainz Jr. | (126) | |
5. Kevin Magnussen | (148) | |
6. Oscar Piastri | (43) | |
7. Esteban Ocon | (98) | |
8. Max Verstappen | (93) | |
9. Lando Norris | (80) | |
10. Zhou Guanyu | (79) | |
Mexico City Grand Prix | 1. Kevin Magnussen | (173) |
2. Sergio Pérez | (164) | |
3. Carlos Sainz Jr. | (141) | |
4. Oscar Piastri | (55) | |
5. Charles Leclerc | (115) | |
6. Max Verstappen | (101) | |
7. Nico HĂŒlkenberg | (87) | |
8. Alexander Albon | (83) | |
9. Yuki Tsunoda | (52) | |
10. Daniel Ricciardo | (91) | |
SĂŁo Paulo Grand Prix | 1. Zhou Guanyu | (104) |
2. Yuki Tsunoda | (70) | |
3. Lewis Hamilton | (99) | |
4. Pierre Gasly | (136) | |
5. Esteban Ocon | (108) | |
6. Fernando Alonso | (90) | |
7. Kevin Magnussen | (179) | |
8. Max Verstappen | (105) | |
9. Charles Leclerc | (117) | |
10. Nico HĂŒlkenberg | (88) | |
Las Vegas Grand Prix | 1. Oliver Bearman | (29) |
2. Charles Leclerc | (135) | |
3. Sergio Pérez | (179) | |
4. Fernando Alonso | (102) | |
5. Max Verstappen | (115) | |
6. Lance Stroll | (70) | |
7. Esteban Ocon | (114) | |
8. Nico HĂŒlkenberg | (92) | |
9. Oscar Piastri | (57) | |
10. Lewis Hamilton | (100) | |
Qatar Grand Prix | 1. Lance Stroll | (95) |
2. Zhou Guanyu | (122) | |
3. Pierre Gasly | (151) | |
4. Charles Leclerc | (147) | |
5. Valtteri Bottas | (130) | |
6. Carlos Sainz Jr. | (149) | |
7. Oscar Piastri | (63) | |
8. Franco Colapinto | (67) | |
9. Lando Norris | (82) | |
10. Fernando Alonso | (103) | |
Abu Dhabi Grand Prix | 1. Zhou Guanyu | (147) |
2. Yuki Tsunoda | (88) | |
3. Kevin Magnussen | (194) | |
4. Esteban Ocon | (126) | |
5. Valtteri Bottas | (140) | |
6. Alexander Albon | (91) | |
7. Sergio Pérez | (185) | |
8. Charles Leclerc | (151) | |
9. Lance Stroll | (97) | |
10. Nico HĂŒlkenberg | (93) |
After doing this, it was fairly easy to sort and display the total points and display the grand champion.
|
|
And the result can be seen below:
P | Driver | Points |
---|---|---|
1 | 20. Kevin Magnussen | 194 |
2 | 11. Sergio Pérez | 185 |
3 | 10. Pierre Gasly | 151 |
4 | 16. Charles Leclerc | 151 |
5 | 55. Carlos Sainz Jr. | 149 |
6 | 24. Zhou Guanyu | 147 |
7 | 77. Valtteri Bottas | 140 |
8 | 31. Esteban Ocon | 126 |
9 | 1. Max Verstappen | 115 |
10 | 14. Fernando Alonso | 103 |
11 | 44. Lewis Hamilton | 100 |
12 | 63. George Russell | 98 |
13 | 18. Lance Stroll | 97 |
14 | 27. Nico HĂŒlkenberg | 93 |
15 | 3. Daniel Ricciardo | 91 |
16 | 23. Alexander Albon | 91 |
17 | 22. Yuki Tsunoda | 88 |
18 | 4. Lando Norris | 82 |
19 | 43. Franco Colapinto | 67 |
20 | 2. Logan Sargeant | 64 |
21 | 81. Oscar Piastri | 63 |
22 | 50. Oliver Bearman | 29 |
As a small observation, with only 3 races ran in this “Season”, Oliver Bearman scores points in about half of the runs. In the few runs I made, Checo Perez, Alexander Albon, Max Verstappen (twice!), and Yuki Tsunoda won the championship before Lando Norris did.
If you want to play with this generator yourself, you can find it available here: Formula 1 Season Advanced Random Simulator
This was a fun experiment and it was nice to brush a bit on my php code. Hope I’ll expand it more soon.